Interactive Brokers Python API (Native)
The Interactive Brokers Python native API is a feature that allows you to automatically trade via Python code (other languages are also supported).
Table of Contents
- IB Python native API vs Third Party Libraries (IBridgePy, IbPy etc)
- Setting up the IB native Python API
IB Python native API vs Third Party Libraries (IBridgePy, IbPy etc)
Interactive Brokers’ native Python API is developed and maintained directly by IB, offering the most stable and reliable connection to their servers. This ensures a smooth trading experience with minimal errors.
On the other hand, third-party libraries like IBridgePy and IbPy, while useful, are not officially supported by IB. A standout third-party option is IB-insync, which enhances the native API’s efficiency by using asynchronous methods for faster communication. It also simplifies data handling, making it a popular choice for traders looking for a more streamlined and responsive trading experience.
Setting up the IB native Python API
System Availability - Before testing, it is a good idea to check the availability of the IBKR system. Trading is available 24 hours a day, 7 days a week with the exception of system reset times described in the table below on the same page.
- Opening Paper Account at IB - If your regular trading account has been approved and funded, you can use your Account Management page to open a Paper Trading Account which lets you use the full range of trading facilities in a simulated environment using real market conditions. [link]
- Downloading the IB native API (Python) provides you with Python scripts that simplify connecting and communicating with the IB client (TWS or IB Gateway), which then links to IB’s servers. Other supported languages include Java, C++, and .NET. Additionally, IB offers integration with Microsoft’s ActiveX framework and DDE for connecting directly to Excel, giving developers a range of options to suit their preferred tools and environments.
- http://interactivebrokers.github.io/ - API version 9.73 or higher is required, as anything before that does not have the necessary Python source files. Python version 3.1 or higher is also required.
- Download the IB client (TWS or IB Gateway) – TWS is the default trading platform provided by Interactive Brokers, offering a comprehensive interface for trading. Alternatively, you can opt for the Interactive Brokers Gateway client, a lightweight solution designed for users who need a simpler, more streamlined connection to the IB server without the full TWS interface. Both options connect directly to IB’s servers and are compatible with the IB API.
In the beginning, it’s recommended to use TWS when testing your script, as it provides a visual confirmation of any activity on your account, making it easier to track and debug your trades. Once you’re comfortable with the setup and your script is running smoothly, you can easily switch to IB Gateway, which is more lightweight and runs without the full graphical interface of TWS.
- Choose your Python IDE
When choosing a Python IDE for working with the IB API, consider these popular options based on your workflow and preferences:
- PyCharm – A powerful and feature-rich IDE, ideal for professional developers. It offers advanced code completion, debugging, and testing features, making it great for complex projects.
- VS Code – A lightweight and highly customizable editor with strong support for Python development. It has a vast marketplace of extensions, including those for Python, and excellent debugging capabilities.
- Jupyter Notebooks – Perfect for those who prefer an interactive environment, especially for testing code snippets, analyzing data, and visualizing trading data in real-time.
- Spyder – A great choice for scientific computing, with an integrated Python environment and excellent tools for code analysis, debugging, and execution, tailored for data-driven workflows.
- Testing Connection
TWS
Testing the connection to the IB API
Log files play a crucial role in diagnosing issues within a custom application. They provide detailed insights into how the application is behaving, making them an invaluable resource for API developers when troubleshooting problems. Beyond aiding developers, these logs can also be uploaded for further analysis by the API Support team, ensuring that any underlying issues are identified and resolved efficiently. This process not only speeds up debugging but also enhances collaboration between developers and support, ultimately leading to more reliable application performance.
- Install the IB API: Make sure you have the Interactive Brokers Python API installed.
1 | $ apt-get install python3-setuptools |
Move the ibapi folder from the pythonclient folder and place it in the directory where we create our API access scripts.
- Enable API Access in TWS: In TWS, go to Global Configuration → API → Settings, and enable the options to allow API connections:
- Write a Basic Python Script: Here’s a simple script to test the connection to TWS or IB Gateway:
1 | from ibapi.client import EClient |
- Run the Script:
- Save the script as
test_ib_connection.py
. - Ensure that TWS or IB Gateway is running on your machine.
- Run the script:
1
python test_ib_connection.py
- Verify Connection: If the connection is successful, you should see an output like:
1
Connected! Next valid order ID: <some_number>
Practical Example: Fetching Market Data
Modify the script: Update your script to request live market data for a specific stock. In this example, we’ll request data for VXX.
To obtain the details needed for the
Contract
object, follow these steps in TWS:- Right-click on the underlying asset in your TWS watchlist.
- Select Description from the context menu.
- A pop-up window will appear, providing all the required information, including symbols, exchange, currency, and other relevant details for constructing the
Contract
object.
This method ensures that you have the correct data for setting up aContract
object in your API scripts.
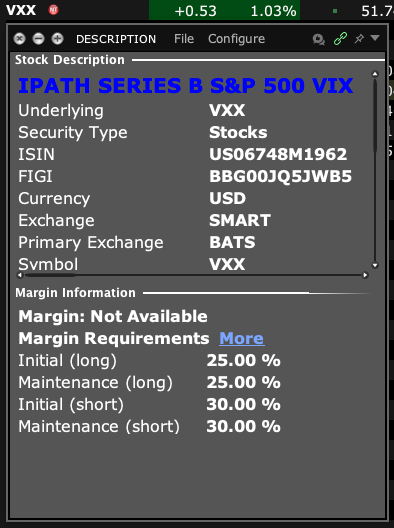
1 | from ibapi.client import EClient |
- Explanation:
- We’ve defined a
Contract
object for VXX stock, specifying the symbol, security type, exchange, and currency. - The method
request_market_data()
sends a request to IB for market data usingreqMktData()
.In our case, we’re requesting live market data (the first parameter), which provides real-time price updates for the specified asset. However, the Interactive Brokers API offers various other data types and request options, all described in the official documentation.
- The
tickPrice()
method receives price updates and prints them to the console.
- Run the Script: Once TWS or IB Gateway is running, execute the script again:
1
python test_ib_market_data.py
- Expected Output: You should see live market data for VXX printed in the console, something like:
1
The current ask price is: <PRICE>
To explore additional options, refer to the Interactive Brokers API documentation, where you’ll find detailed descriptions of each feature, along with the required parameters for requesting them.
With this information, you can easily extend your script beyond live market data to cover more advanced use cases like backtesting with historical data, scanning the markets, or receiving financial news.
Client
If a certain feature or operation is not available in the TWS, it will not be available on the API side either! [link]
The TWS/ IB Gateway is designed to accept up to 50 messages per second coming from the client side. Anything coming from the client application to the TWS/ IB Gateway counts as a message (i.e. requesting data, placing orders, requesting your portfolio… etc.). This limitation is applied to all connected clients in the sense where all connected client applications to the same instance of TWS/ IB Gateway combined cannot exceed this number. On the other hand, there are no limits on the amount of messages the TWS can send to the client application. [link]
Q | A |
---|---|
Is it possible to have multiple API connections to a single TWS session (or IB gateway) at the same time? | Yes, using different client IDs for each API program. |
Is it possible to run multiple TWS sessions on the same API-enabled machine? | Yes, using different socket port numbers for each API program. |
Should API functions be called immediately after the connect function is called? | No, there should be a short pause as the program waits for automatic callbacks such as nextValidId to indicate the completion of the initial connection. |
Sources
This article is just an outline of the possibilities and it is more than advisable to focus on very detailed documentation afterwards.
- https://www.interactivebrokers.com/campus/ibkr-api-page/twsapi-doc/#api-introduction - I strongly recommend reading the entire documentation
- https://www.interactivebrokers.com/campus/trading-course/python-tws-api/
- https://www.interactivebrokers.com/campus/trading-lessons/python-receiving-market-data/
- https://www.ibkrguides.com/clientportal/aboutpapertradingaccounts.htm